随着微服务的流行,服务和服务之间的稳定性变得越来越重要。 Sentinel 以流量为切入点,从流量控制、熔断降级、系统负载保护等多个维度保护服务的稳定性。
Sentinel特征
丰富的应用场景: Sentinel 承接了阿里巴巴近 10 年的双十一大促流量的核心场景,例如秒杀(即突发流量控制在系统容量可以承受的范围)、消息削峰填谷、实时熔断下游不可用应用等。
完备的实时监控: Sentinel 同时提供实时的监控功能。您可以在控制台中看到接入应用的单台机器秒级数据,甚至 500 台以下规模的集群的汇总运行情况。
广泛的开源生态: Sentinel 提供开箱即用的与其它开源框架/库的整合模块,例如与 Spring Cloud、Dubbo、gRPC 的整合。您只需要引入相应的依赖并进行简单的配置即可快速地接入 Sentinel。
完善的 SPI 扩展点: Sentinel 提供简单易用、完善的 SPI 扩展点。您可以通过实现扩展点,快速的定制逻辑。例如定制规则管理、适配数据源等。
Sentinel控制台
Sentinel
提供了两种使用方式,包括使用阿里云提供的服务,当初推广的时候是免费的
在线控制台
下载地址
项目使用Sentinel(阿里云控制台)
ahas文档
引入ahas依赖
1
2
3
4
5
| <dependency>
<groupId>com.alibaba.csp</groupId>
<artifactId>ahas-sentinel-client</artifactId>
<version>1.6.4</version>
</dependency>
|
添加bean
1
2
3
4
5
6
7
8
9
| @Bean
public FilterRegistrationBean sentinelFilterRegistration() {
FilterRegistrationBean registration = new FilterRegistrationBean();
registration.setFilter(new CommonFilter());
registration.addUrlPatterns("/*");
registration.setName("sentinelCommonFilter");
registration.setOrder(1);
return registration;
}
|
项目启动
1
| java Dproject.name=AppName -Dahas.license=<License> -jar xxxx.jar
|
使用自己搭建的控制台
启动Sentinel控制台
1
| java -Dserver.port=8081 -Dcsp.sentinel.dashboard.server=localhost:8081 -Dproject.name=sentinel-dashboard -jar sentinel-dashboard-1.7.2.jar
|
PS:用户名密码都是sentinel
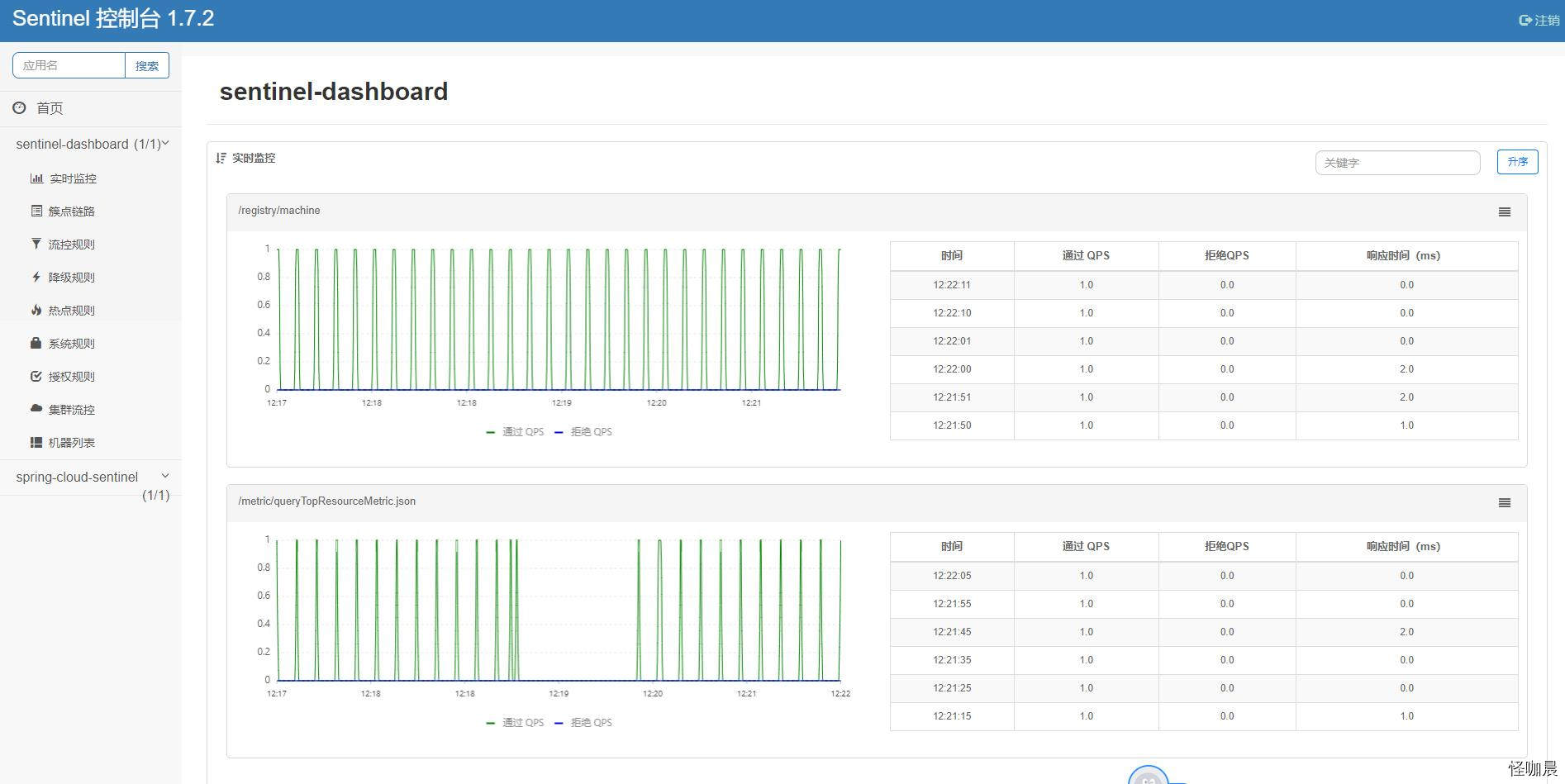
引入依赖
1
2
3
4
| <dependency>
<groupId>com.alibaba.cloud</groupId>
<artifactId>spring-cloud-starter-alibaba-sentinel</artifactId>
</dependency>
|
简单示例
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
| @SpringBootApplication
public class SentinelApplication {
public static void main(String[] args) {
SpringApplication.run(SentinelApplication.class, args);
}
}
@RestController
public class IndexController {
@Autowired
private SayHelloService sayHelloService;
@GetMapping
public String sayHello(String name) {
return sayHelloService.sayHello(name);
}
}
public interface SayHelloService {
String sayHello(String name);
}
@Slf4j
@Service
public class SayHelloServiceImpl implements SayHelloService {
@SentinelResource("sayHello")
public String sayHello(String name) {
log.info(Thread.currentThread().getName());
try {
Thread.sleep(1000);
}catch (Exception e){
e.printStackTrace();
}
return "hello ".concat(name).concat("!");
}
}
|
application.yaml配置
1
2
3
4
5
6
7
| spring:
application:
name: spring-cloud-sentinel
sentinel:
transport:
port: 8081
dashboard: localhost:8081
|
@SentinelResource
注解用来标识资源是否被限流、降级。上述例子上该注解的属性 sayHello 表示资源名。
规则配置
流控规则》新增流控规则
- 输入资源名,和代码中
@SentinelResource
注解的值对应 - 根据对应需求选择阈值类型,分为
QPS
及线程数
- 设置阈值类型对应的数值,比如同一时间内只允许有一个线程访问,可以选择为
线程数
,单机阈值
为1
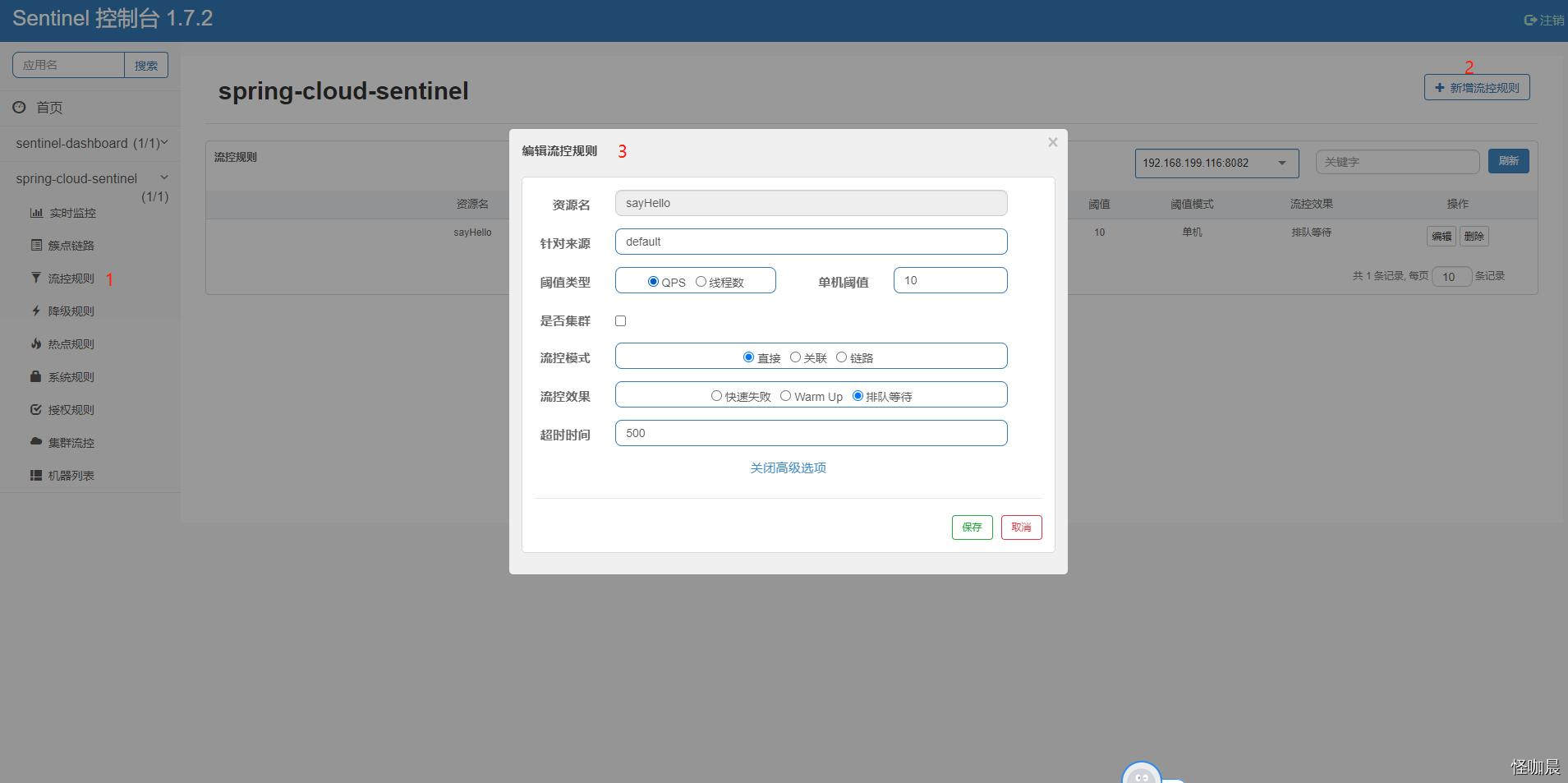
线程测试
测试环境:阈值类型为线程数
,单机阈值为10
,jMeter设置线程为20
,失败率为50%
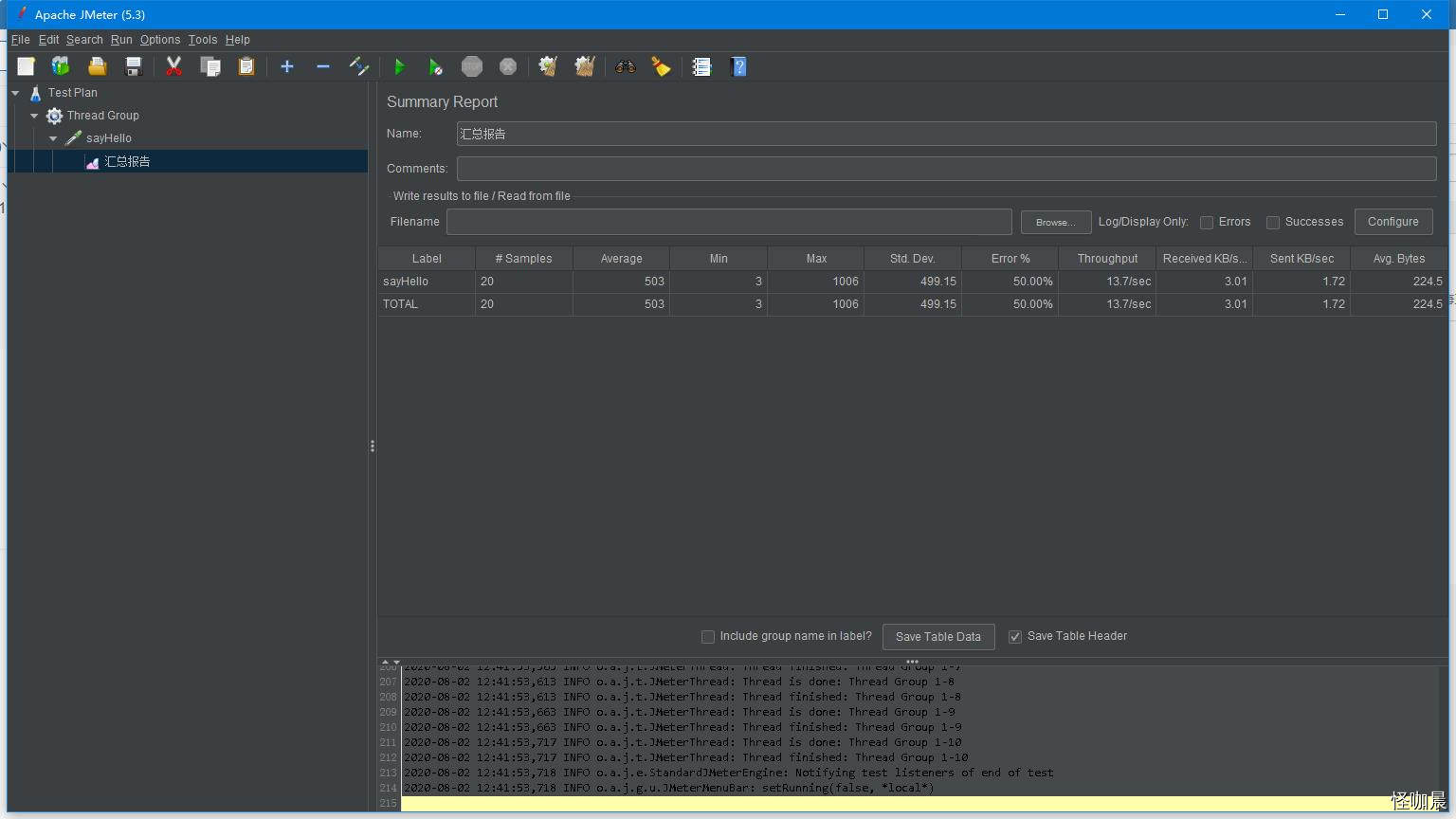
QPS测试
测试环境:阈值类型为QPS
,单机阈值为10
,jMeter设置线程为20
,失败率为40%
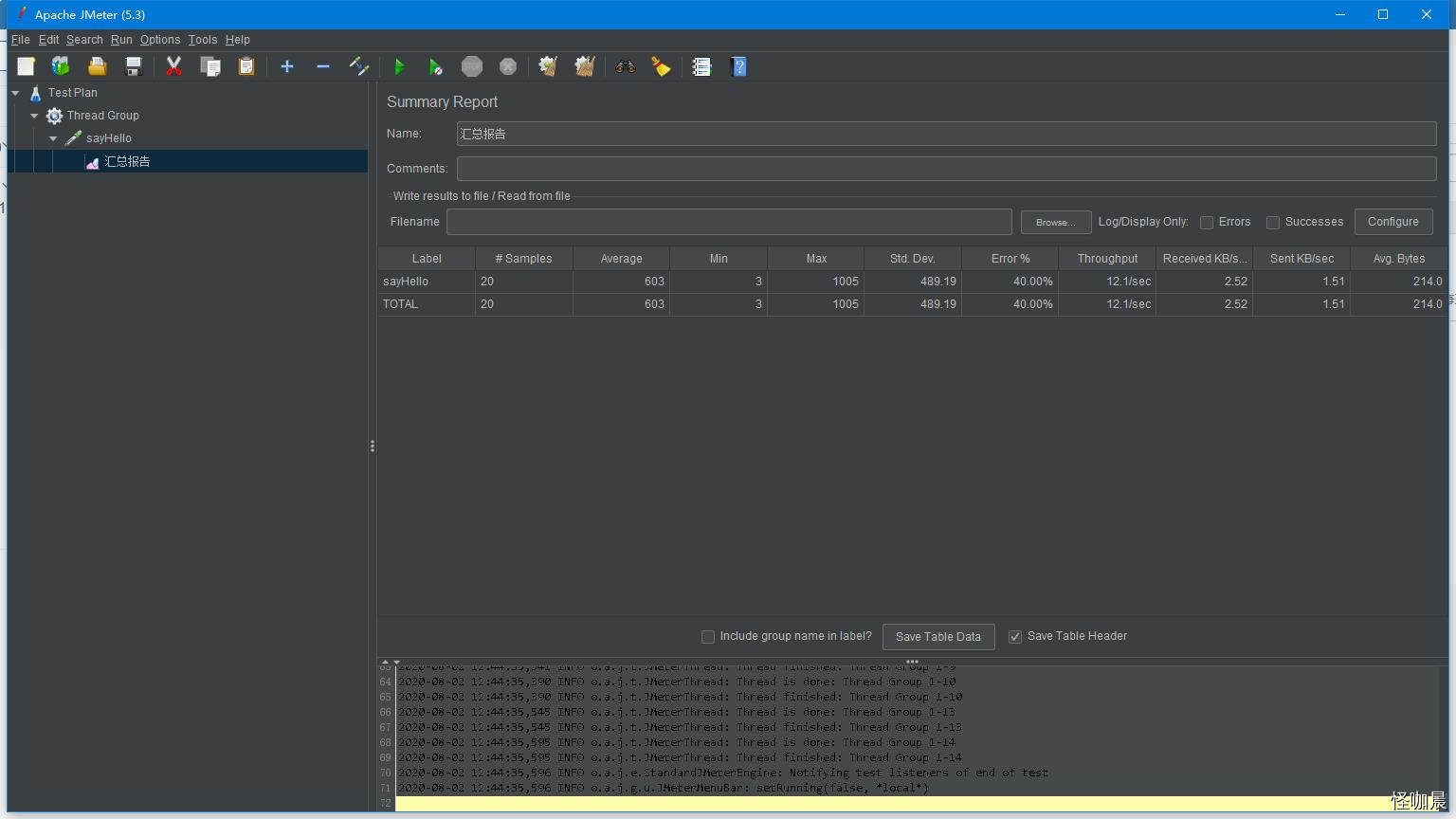
代码地址